Remove the Last Character from a String in JavaScript
In this guide, I will walk you through various methods to easily remove the last character from a string in JavaScript.
Using the slice() method
To remove the last character from a string, we can use the slice()
method. The slice()
method extracts a part of a string and returns it as a new string without modifying the original string. This method takes two arguments: the start index and the end index.
The extracted string starts from the start index and goes up to, but doesn’t include, the character at the end index.
The slice()
method supports negative indexing. When using negative indexes, the position is counted from the end of the string. For example, -1
represents the last character of the string, -2
represents the second last character, and so on.
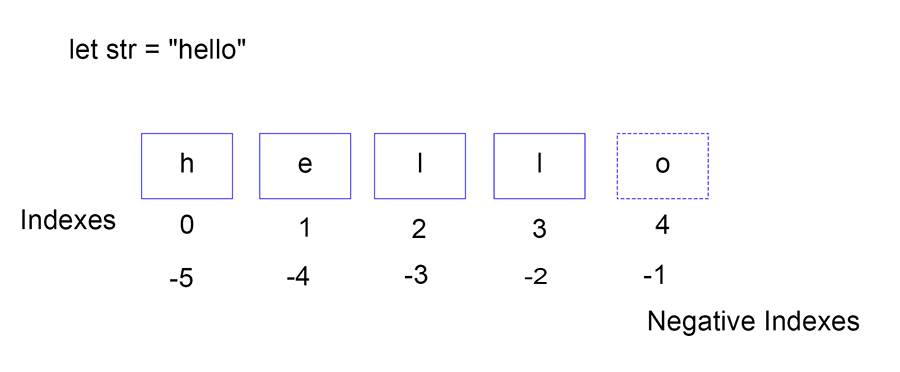
To remove the last character, we can pass 0
as the start index and -1
as the end index.
For example:
let str = "hello";
let newStr = str.slice(0, -1);
console.log(newStr); // Output: hell
Similarly, to remove the last two characters, we can pass 0
as the start index and -2
as the end index.
For example:
let str = "hello";
let newStr = str.slice(0, -2);
console.log(newStr); // Output: hel
So, we can say to remove the last n
characters, we have to pass 0
as the start index and -n
as the end index.
See Also: Remove the First and the Last Character from a String in JavaScript
Using the substring() method
We can also use the substring()
method to remove the last character from a string. This method takes two arguments: the start index and the end index.
To remove the last character, we can pass 0
as the start index and string.length - 1
as the end index. This is because string indexes start from 0
. We need to subtract 1
from the length of the string to get the index of the last character.
For example:
let str = "hello";
let newStr = str.substring(0, str.length - 1);
console.log(newStr); // Output: hell
Unlike the slice()
method, the substring()
method doesn’t support negative indexing.
The substring()
method also returns a new string without modifying the original string.
Using the replace() method
We use replace()
method to replace specified values or patterns in a string with new values. However, we can also use replace()
method to remove the last character from a string.
The replace()
method takes two arguments: the first is the pattern to search for, and the second is the string to replace it with.
For example:
let str = "hello";
let newStr = str.replace(/.$/, "");
console.log(newStr); // Output: hell
Let’s break down the regular expression /.$/
/
Forward slashes indicate the start and the end of the regular expression.
.
The period or dot is a metacharacter that matches any character except for a newline character \n
.
$
The dollar sign character is an anchor that matches the end of the string.
The regular expression /.$/
matches any character at the end of the string.
In the above example, we use the regular expression /.$/
to match any character that appears at the end of the string. We then use the replace()
method to replace this character with an empty string, effectively removing the last character from the string.
Note: It is important to note that the regular expression /.$/
we used in the above example does not consider the presence of newline character \n
at the end of the string. This means that if the string ends with a newline character, it will not be matched and will not be replaced with an empty string.
To match and remove a newline character \n
that appears at the end of the string, we can pass the regular expression /.$|\n$/
as the search pattern.
This regular expression uses the |
character to specify an “or” condition, meaning that it will match either any character at the end of the string (using .$
) or a newline character at the end of the string (using \n$
).
We can then use the replace()
method with an empty string ""
as the replacement value to remove the matched character from a string.
For example:
let str = "hello\n";
let newStr = str.replace(/.$|\n$/, "");
console.log(newStr); // Output: hello
let newStrTwo = str.replace(/.$/, "");
console.log(newStrTwo); // Output: hello
In the example above, the value of newStr
and newStrTwo
may appear identical when printed to the console. However, they are different. The string newStr
doesn’t contain a newline character \n
a the end, while newStrTwo
does. This difference can be observed by concatenating another string to both variables and printing the result to the console.
Here, is an example:
let str = "hello\n";
let newStr = str.replace(/.$|\n$/, "");
console.log(newStr + " bros");
let newStrTwo = str.replace(/.$/, "");
console.log(newStrTwo + " world");
Output:
hello bros
hello
world
We can use a regular expression with specific logic to remove a particular character from the end of the string.
Remove the last character only if it is a whitespace
In the example below, we remove the last character from a string only if it is a whitespace character.
// String with tab character \t at the end
let a = "hello\t";
let x = a.replace(/\s$/, "");
console.log(x); // Output: hello
// String with empty space character at the end
let b = "hello ";
let y = b.replace(/ $/, "");
console.log(y); // Output: hello
The regular expression \s
matches any whitespace characters like a tab, space, carriage return, line feed, or form feed. The regular expression /\s$/
matches any whitespace character at the end of the string.
If you want to match only the space character at the end of the string, use the regular expression / $/
as the search pattern.
Remove the last character only if it matches a specific character
In the example below, we remove the last character from the string only if it matches a specific character we want to remove.
// Removes the last character only if it is e
let a = "apple";
let x = a.replace(/e$/, "");
console.log(x); // Output: appl
// Removes the last character only if it is a comma
let b = "apple, banana, mango,";
let y = b.replace(/,$/, "");
console.log(y); // Output: apple, banana, mango
// Removes the last character only if it is forward slash /
let c = "hello/";
let z = c.replace(/\/$/, "");
console.log(z); // Output: hello
If the last character is a metacharacter
A metacharacter is a character that has a special meaning and is used to define a pattern that the regular expression engine uses to match the text.
If you want to match these characters literally in a regular expression, you need to escape them with a backslash (\
). This tells the regular expression engine to treat the metacharacter as a regular character and match it literally in the text.
For example, if you want to match the period character (.
) literally in a regular expression, you would need to escape it with a backslash like this: \.
. This tells the regular expression engine to match the period character literally instead of treating it as a metacharacter that matches any character.
In the example below, we will remove the specific metacharacter we want to remove at the end of the string.
// Removes the last character if it is a period or dot character
let a = "I am fine.";
let x = a.replace(/\./, "");
console.log(x); // Output: I am fine
// Removes the last character if it is a forward slash / character
let b = "javascript/";
let y = b.replace(/\//, "");
console.log(y); // Output: javascript
Below is a list of all the meta characters that need escaping if you need to match them in a regular expression:
- Backslash
\
- Caret
^
- Dollar Sign
$
- Period or dot
.
- Vertical bar or pipe symbol
|
- Question mark
?
- Asterisk
*
- Plus sign
+
- Opening round bracket
(
- Closing round bracket
)
Using the split() and join() method
We can also use the split()
and join()
methods to remove the last character from a string. Here is how:
- Use the
split()
method with an empty string as the separator to split the string into an array of individual characters. - Use the
pop()
method to remove the last element from the array. - Use the
join()
method with an empty string as the separator to join the elements of the array back into a string.
Here, is an example:
let str = "hello";
let str_as_array = str.split("");
console.log(str_as_array); // Output: ['h', 'e', 'l', 'l', 'o']
str_as_array.pop();
let finalString = str_as_array.join("");
console.log(finalString); // Output: hell
Using the for loop
We can use the for
loop to remove the last character from a string.
Here, is an example:
let str = "hello";
let newStr = "";
for (let i = 0; i < str.length - 1; i++) {
newStr += str[i];
}
console.log(newStr); // Output: hell
In the above example, the for
loop iterates from 0
to str.length - 1
, which means that it will iterate over all the characters in the string except for the last one. This is because the loop condition i < str.length - 1
will be false when i
is equal to str.length - 1
, so the loop will stop before the last character is reached.
Initially, we assign a variable newStr
with an empty string. During each iteration of the loop, we access the character at the current index of the string str
and concatenate it to newStr
. As a result, we build a new string newStr
that contains all the characters from the original string except the last one.
Conclusion:
The slice()
method provides a clean and elegant way to remove the last character from the string.
However, if you need to use specific logic to remove the last character from a string, you can use the replace()
method. This method allows you to search for a specific pattern in a string and replace it with a new value.
You can use any of the methods we discussed above you feel comfortable with to remove the last character from the string.