Convert a List to a Comma-Separated String in Python
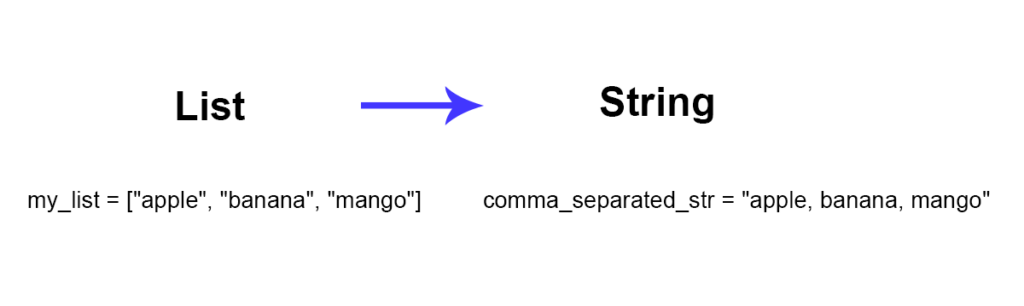
To convert a list to a comma-separated string, we can use the join()
method. This method concatenates the elements of an iterable (such as a list, tuple, or string) into a single string using a specified separator.
The syntax for the join()
method is as follows:
separtor_string.join(iterable)
To convert a list to a comma-separated string, we can call the join()
method on the separator ", "
and pass the list as the argument.
Here is an example:
my_list = ["apple", "banana", "mango"]
comma_separated_str = ", ".join(my_list)
print(comma_separated_str) # Output apple, banana, mango
In the above example, I used a comma and a space ", "
as the separator. However, you can use any character as a separator. Additionally, the iterable passed to the join()
method doesn’t have to be a list. It can be any iterable object such as a tuple, string, or dictionary.
If the list contains non-string elements (such as integers, or floats), we will need to convert them to strings before calling the join()
method. We can do this using a list comprehension or the map function.
Here is an example:
my_list = ["MrBeast", 6000, 1.91]
# Using map
comma_separated_str = ", ".join(map(str, my_list))
print(comma_separated_str) # Output MrBeast, 6000, 1.91
# Using list comprehension
comma_separated_str2 = ", ".join([str(x) for x in my_list])
print(comma_separated_str2) # Output MrBeast, 6000, 1.91