Remove the Last Character from a String in Python
When working with strings in Python, removing unwanted trailing characters like whitespace, commas, periods, slashes, and newlines is often necessary.
This guide will explore various methods to remove the last character from a string effectively. We will discuss the following methods:
- Using string slicing
- Using rstrip() method
- Using for loop
- Using regular expression
#1 Using string slicing
In Python, you can use string slicing to extract a portion of the string.
The basic syntax for string slicing is:
string[start:stop:step]
Where,
start
is the index where the slice begins.stop
is the index where the slice ends (exclusive).step
is the step size.
Here’s a basic example of string slicing:
str = "hello"
new_str = str[1:3]
print(new_str) # el
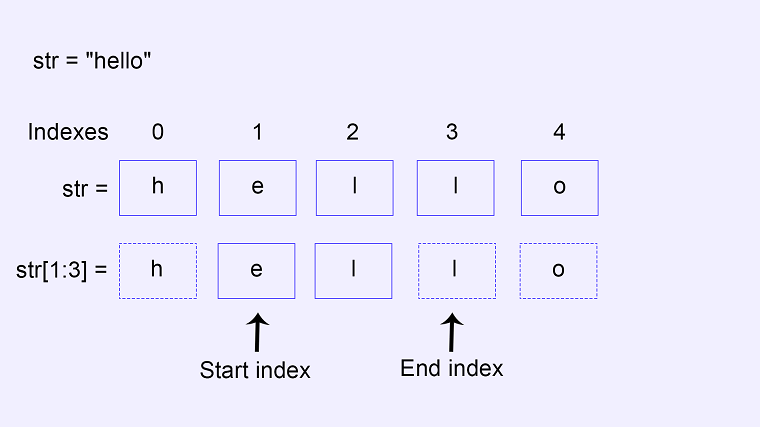
The string slicing extracts a part of the string and returns it as a new string without modifying the original string.
The extracted string starts from the start index and goes up to, but doesn’t include, the character at the end index.
The string slicing also supports negative indexing. When using negative indexes, the position is counted from the end of the string. For example, -1
represents the last character of the string, -2
represents the second last character, and so on.
The above example can be rewritten using negative indexing as follows:
str = "hello"
new_str = str[1:-2]
print(new_str) # el
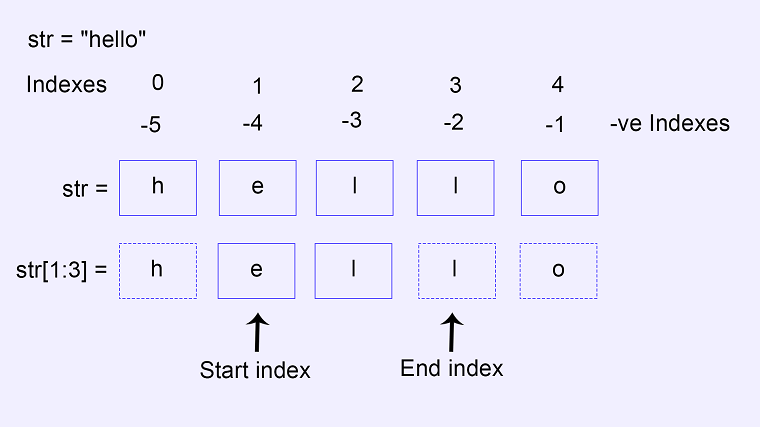
Removing the last character
To remove the last character from the string, you can pass 0
as the start index and -1
as the end index.
For example:
str = "hello"
new_str = str[0:-1]
print(new_str) # hell
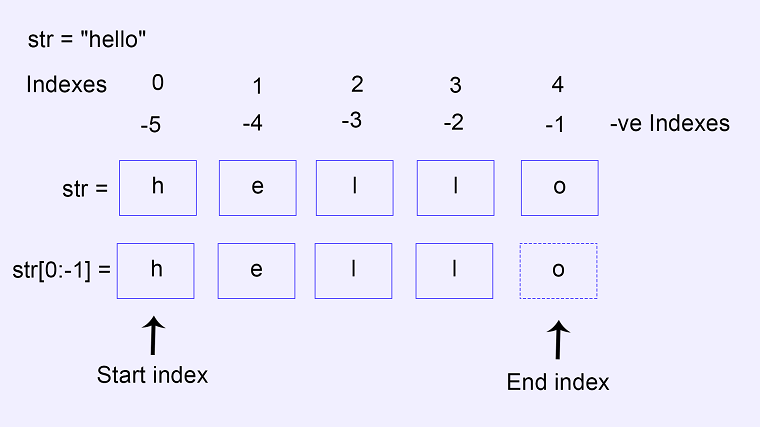
If you omit the start index, the slicing will start from the beginning of the string, including the first character.
For example:
str = "hello"
new_str = str[:-1]
print(new_str) # hell
If you want to remove the last two characters, pass -2
as the end index.
For example:
str = "hello"
new_str = str[:-2]
print(new_str) # hel
If you omit the end index, the slicing will go up to the end of the string.
For example:
str = "hello"
new_str = str[1:]
print(new_str) # ello
#2 Using rstrip() method
The rstrip()
method removes trailing characters (characters at the end) from a string. It returns a new string with the trailing characters removed, leaving the original string unchanged.
The syntax for rstrip()
method is:
string.rstrip(characters)
where,
string
: The string on which the rstrip()
method is called on.
characters
: An optional parameter specifying the set of characters to be removed from the right of the string. If not provided, it removes all trailing whitespaces (spaces, tabs, and newline characters).
Removing trailing whitespace
The code below removes all trialing whitespaces from the string:
str = "Python is awesome! "
new_str = str.rstrip()
print(new_str) # Python is awesome!
The whitespace is the default parameter. There is no need to explicitly pass any parameters.
Removing specific trailing characters
The code below removes the trailing character if it is an exclamation mark. However, feel free to tweak the code to remove any trailing character you wish.
str = "Python is awesome!!!"
new_str = str.rstrip('!')
print(new_str) # Python is awesome
Removing the trailing characters if they are comma, period, or exclamation point
The code below removes all the occurrences of the last characters if they are commas, periods, or exclamation points:
str = "Python is awesome...!!!"
new_str = str.rstrip('!.,')
print(new_str) # Python is awesome
If you want to dynamically assign the value of the last character, you can achieve this by using the following code:
str = "Python is awesome...!!!"
new_str = str.rstrip(str[-1])
print(new_str) # Python is awesome…
The str[-1]
refers to the last character of the string str
.
Additionally, it is important to remember that the rstrip()
method removes all instances of the specified characters from the end of the string, not just the first occurrence.
#3 Using for loop
You can remove the last character from a string using the for
loop by iterating through the characters of the string and creating a new string without the last character.
Here’s an example:
str = "Python is awesome!"
new_str = ""
# Iterate up to the second last character
for i in range(len(str) - 1):
new_str += str[i]
print(new_str) # Python is awesome
In this example, the for
loop iterates through the characters of the string str
except for the last one, and each character from the original string str
is appended to the new_str
using string concatenation (+=
). The result is a new string without the last character.
#3 Using regular expression
A regular expression (regex) is a sequence of characters that define a search pattern. It is a powerful tool used for matching, locating, and manipulating text.
You can use regular expressions to remove the last character from the string. In this approach, we replace the last character of the string with an empty string.
Here’s an example:
import re
def remove_last_character(str):
return re.sub(r'.$', '', str)
print(remove_last_character("hello")) # hell
First, you must import the re
module to use regular expressions in Python.
The re.sub()
method is a part of the re
(regular expression) module and is used for replacing substrings that match a specified pattern with the replacement string.
The basic syntax for the re.sub()
method is as follows:
re.sub(pattern, replacement, string, count=0, flags=0 )
pattern
: This is the regular expression pattern you want to search for in a string.
replacement
: This is the string that will replace the matched pattern.
string
: This is the input string (original string) where the pattern will be searched for and replaced.
count
(optional): If specified, it limits the number of replacements. The default is 0, meaning all occurrences will be replaced.
flags
(optional): Additional flags that modify the behavior of the regular expression. For example, re.IGNORECASE
can be used to perform a case-insensitive search.
In the above example, .$
is a regular expression pattern. Here,
.
: This is a special character in a regular expression that matches any character except for a newline. In other words, it matches any single character, whether it is a letter, digit, punctuation mark, or whitespace.
$
: This is another special character in regular expressions that asserts the position at the end of the line. It doesn’t match any character itself; instead, it matches the position right before the end of the string.
So, when you combine .$
, the pattern matches any single character (.
) that appears at the end of the string $
. In other words, it matches the last character in a string. In the context of your, re.sub(r'.$', '', str)
usage, it is removing the last character from the input string by replacing it with an empty string.
If you want to remove the last character, including a newline character if it is present, you can modify the regular expression pattern to .$|\n$
.
.$
: Matches any character except a newline at the end of the string.|
: This is a logical OR operator.\n$
: Matches a newline character at the end of the string.
Removing specific trailing characters
The code below removes the last character from a string if it is an exclamation mark:
import re
def remove_last_character(str):
return re.sub(r'!$', '', str)
print(remove_last_character("hello!")) # hello
print(remove_last_character("python")) # python
Let’s break down the regular expression !$
:
!
: Matches an exclamation mark$
: Matches the end of the string.
Together, the pattern !$
matches the last character if it is an exclamation mark.
Feel free to tweak the above code to remove any trailing character you wish. However, keep in mind that in regular expression, some characters are reserved and hold special meanings. To treat these characters as ordinary literals within a regex pattern, you will need to escape them with a backslash.
For instance, to remove the trailing plus sign (+), you would escape it as follows: \+$
Here’s a list of special characters that need escaping:
.
dot*
asterisk+
plus?
question mark|
pipe symbol(OR operator)^
caret(
and )
parentheses[
and ]
square brackets{
and }
curly braces\
forward slash
Remove the trailing character if it is a period, comma, semicolon, or forward slash
The code below removes the last character if it is a period, comma, semicolon, or forward slash:
import re
def remove_last_character(str):
return re.sub(r'[.,;/]$', '', str)
print(remove_last_character("hello.")) # hello
print(remove_last_character("apple,")) # apple
print(remove_last_character("python;")) # python
print(remove_last_character("awesome/")) # awesome
In this example, the regular expression [.,;/]$
is used:
[.,;/]
: Matches any one of the characters inside the square brackets (.
or ,
or ;
or /
). In regular expressions, square brackets ([]
) are used to define a character class. A character class allows you to specify a set of characters, and the pattern will match any single character from that set.
$
: Matches the end of the string.
So, the pattern [.,;/]$
effectively matches the last character of the string only if it is one of the specified characters.
Feel free to add or remove any character you wish inside the character class to remove the desired trailing character from the list.
Remove all occurrences of the specific trailing period, comma, semicolon, or forward slash
The code below removes all the occurrences of period, comma, semi-colon, or forward slashes from the end of the string if it present:
import re
def remove_last_character(str):
return re.sub(r'[.,;/]+$', '', str)
print(remove_last_character("hello..;;,,")) # hello
print(remove_last_character("apple,")) # apple
print(remove_last_character("python;")) # python
print(remove_last_character("awesome/")) # awesome
print(remove_last_character("home")) # home
In this example, the regular expression [.,;/]+$
is adjusted to match one or more occurrences of characters from the specified list. The +
quantifier captures one or more characters consecutively from the character class.
Conclusion
In summary, the choice of method for removing the last character from the string ultimately hinges on your specific needs and preferences.
For a straightforward and easy-to-understand approach, string slicing stands out as a simple yet effective technique.
If your goal is to remove all instances of a particular trailing character, the rstrip()
method offers a concise solution
On the other hand, if you seek greater control and flexibility in tailoring your string manipulation, leveraging regular expressions provides a powerful and versatile option.
Remember, the most suitable method depends on the unique requirement of your task, feel free to explore and choose the technique that aligns best with your programming objectives.