Remove the First Character from a String in Python
When working with strings in Python, you may encounter undesirable leading characters like dollar signs, forward or backward slashes, and extra whitespaces that can interfere with your intended string operations. It becomes essential to eliminate these unwanted characters.
In this guide, I will instruct you to remove such preceding characters from your string. We will explore the following methods:
- Using slicing
- Using lstrip() method
- Using replace() method
- Using list
- Using for loop
- Using regular expression
# Using slicing
Before diving into removing characters, let’s understand slicing. Slicing is a powerful feature in Python that allows you to extract a portion of a sequence, such as a string.
The basic syntax of slicing is:
string[start:stop:step]
Where,
start
is the index where the slice begins.stop
is the index where the slice ends (exclusive).step
is the step size.
Here’s a basic example of string slicing:
str = "hello"
new_str = str[1:3]
print(new_str) # el
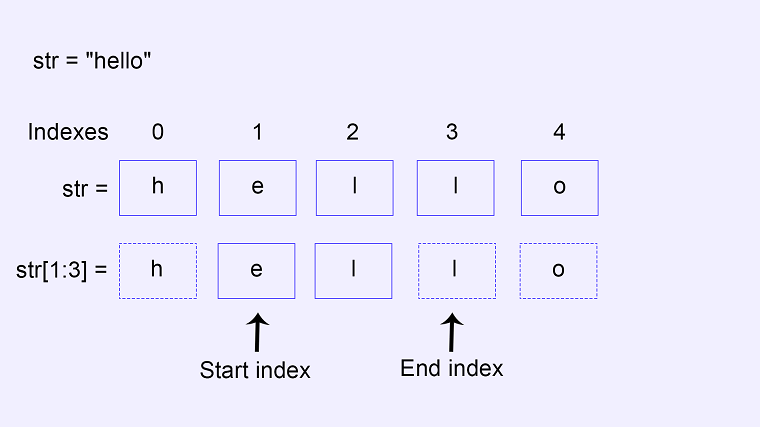
The string slicing extracts a part of the string and returns it as a new string without modifying the original string.
The extracted string starts from the start index and goes up to, but doesn’t include, the character at the end index.
Using slicing to remove the first character
In Python, string indexing starts at position 0
. Therefore, to remove the first character from a string, simply specify 1
as the start index.
For example:
str = "hello"
new_str = str[1:]
print(new_str) # ello
By omitting the end index, you allow the slicing operation to continue until the end of the string.
# Using lstrip() method
The lstrip()
method in Python removes leading characters (characters at the beginning) from a string. However, it doesn’t specifically remove the first character; instead, it removes all the leading characters that match the provided argument.
Here’s an example:
str = "hello"
new_str = str.lstrip('h')
print(new_str) # ello
Let’s take a look at another example:
str = "ooze"
# The lstrip() method removes all leading 'o' characters from the string
new_str = str.lstrip('o')
print(new_str) # ze
If you want to remove just the first character, you can use string slicing or other methods.
Removing leading whitespaces using lstrip() method
The lstrip()
method removes leading whitespaces by default, so you don’t need to specify any parameters.
For example:
str = " hello"
new_str = str.lstrip()
print(new_str) # hello
# Using replace() method
In Python, the replace()
method is a built-in string method used to replace occurrences of a specified substring with another substring.
The general syntax of the replace()
method is as follows:
new_string = orignal_string.replace(old_substring, new_substring, count)
Here’s a breakdown of the parameters:
original_string
: This is the string in which you want to replace occurrences of a substring.
old_substring
: This is the substring that you want to replace.
new_substring
: This is the substring that will replace occurrences of the old substring.
count
(optional): This parameter specifies the maximum number of occurrences to replace. If not specified, all occurrences will be replaced.
The replace()
method returns a new string with the specified replacements. It is important to note that strings in Python are immutable, so the replace()
method does not modify the original string but instead creates and returns a new string with the specified replacement.
Here’s a simple example:
str = "I love JavaScript"
new_str = str.replace("JavaScript", "Python", 1)
print(new_str) # I love Python
In this example, the replace()
method is used to replace only the first occurrence of "JavaScript"
with "Python"
in the original string "str"
.
Using replace() method to remove the first character
You can use the replace()
method to remove the first character from a string by replacing it with an empty string.
Here’s an example:
str = "hello"
new_str = str.replace(str[0], "", 1)
print(new_str) # ello
In this example, str[0]
represents the first character of the string, and we use the replace()
method to replace it with an empty string ""
. The third parameter 1
ensures that only the first occurrence is replaced. As a result, the modified string new_str
does not include the first character of the original string.
# Using list
You can remove the first character from a string using a list by converting the string to a list of characters, removing the first character, and then joining the list back into a string.
Here’s an example:
str = "hello"
# Convert a string to a list of characters
char_list = list(str)
# Remove the first character at index 0
char_list.pop(0)
# Join the list back into a string
new_str = ''.join(char_list)
print(new_str) # ello
# Using for loop
You can remove the first character from a string using a for
loop by iterating through the characters of the string starting from the second character and creating a new string.
Here’s an example:
str = "Python"
new_str = ""
# Using a for loop to remove the first character
for i in range(1, len(str)):
new_str += str[i]
print(new_str) # ython
In this example, the loop starts at index 1
(the second character) and iterates up to the length of the string len(str)
. Within the loop, every character from the original string is added to the new string new_str
. The outcome is a modified string new_str
that excludes the initial character.
# Using regular expression
Regular expressions (regex or regexp) in Python are a very powerful tool for pattern matching and text manipulation. They are implemented through the re
module in Python.
You can use the re.sub()
method to remove the first character from a string using a regular expression.
Here’s an example:
import re
str = "hello"
# Matches the first character in the string
pattern = r'^.'
new_str = re.sub(pattern, '', str)
print(new_str) # ello
The re.sub()
method is a part of the re
(regular expression) module and is used for replacing substrings that match a specified pattern with the replacement string.
The basic syntax for the re.sub()
method is as follows:
re.sub(pattern, replacement, string, count=0, flags=0 )
pattern
: This is the regular expression pattern you want to search for in a string.
replacement
: This is the string that will replace the matched pattern.
string
: This is the input string (original string) where the pattern will be searched for and replaced.
count
(optional): If specified, it limits the number of replacements. The default is 0, meaning all occurrences will be replaced.
flags
(optional): Additional flags that modify the behavior of the regular expression. For example, re.IGNORECASE
can be used to perform a case-insensitive search.
In this example, '^.'
is a regular expression pattern. Here,
^
: Anchors the position at the beginning of the string..
: Matches any character except for a new line. In other words, it matches any single character, whether it is a letter, digit, punctuation mark, or whitespace.
When combined as '^.'
in the context of re.sub(pattern, '', str)
, the pattern effectively matches the first character of the string, subsequently removing it through the replacement with an empty string.
If you want to remove the last character, including a newline character if it is present, you can modify the regular expression to '^.|^\n'
.
^.
: Matches any character except for a newline at the beginning of the string.|
: This is a logical OR
operator^\n
: Matches a newline character at the beginning of the string.
Removing the specific leading character
The code below removes the first character from a string only if it is an exclamation mark:
import re
def remove_first_character(str):
pattern = r'^!'
return re.sub(pattern, '', str)
new_str = remove_first_character("!hello")
another_str = remove_first_character("python")
print(new_str) # hello
print(another_str) # python
Let’s break down the regular expression '^!'
:
^
: Anchors the position at the beginning of the string.!
: Matches an exclamation mark.
Together, the pattern '^!'
matches an exclamation mark if it is the first character of a string.
Feel free to tweak the code to remove any leading character you wish. However, keep in mind that in regular expression, some characters are reserved and hold special meanings. To treat these characters as ordinary literals within a regex pattern, you will need to escape them with a backslash.
For instance, to remove the leading plus sign (+
), you will lead to escape it as follows: '^\+'
Here’s a list of special characters that need escaping:
.
dot*
asterisk+
plus?
question mark|
pipe symbol(OR operator)^
caret(
and )
parentheses[
and ]
square brackets{
and }
curly braces\
forward slash
Conclusion
In conclusion, we have explored various methods to remove the last character from a string in Python, each with its own strengths and use cases.
Slicing provides a concise and elegant solution, allowing for quick removal of the first character.
The lstrip()
method proves handy when dealing with strings and removing a specific character from the beginning of the string.
The replace()
method, although primarily designed for string manipulation, can also be employed creatively to eliminate the first character from a string.
Using a list and for
loop allows for versatile and adaptable string manipulation. This approach offers flexibility in handling diverse data patterns and provides a robust solution for scenarios with varying string structures, catering to different data types while addressing specific manipulation challenges.
For those comfortable with regular expressions, this powerful tool offers a concise and expressive way to achieve the desired outcome. While it might seem overkill for simple tasks, regular expressions shine in scenarios where more advanced pattern matching is required.
Ultimately, the choice of method depends on the specific requirements of your task, as well as personal preference and code readability. Whichever approach you choose, mastering multiple methods enhances your proficiency as a Python programmer, enabling you to tackle diverse challenges with confidence.